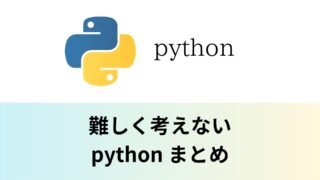
目標
(pythonの)「文字列」について、様々な作成方法を理解して利用できる。
様々な作成方法
- 「’ ‘」シングルクォーテーションでの作成方法
- 「” “」ダブルクォーテーションでの作成方法
- 「”’ ”’」三連単一引用符での作成方法
- 「””” “””」三連二重引用符での作成方法
- 「’ ‘」や「” “」でのエスケープシーケンスの利用方法
- その他の注意点
(pythonの)「文字列」の作成方法
(pythonの)「文字列」の作成方法
(pythonの)「文字列」の作成方法の概要
「文字列」は「’ ‘」シングルクォーテーションや「” “」ダブルクォーテーションを利用して記述します。
また、複数行にまたがる文字列を利用する場合は、三連単一引用符「”’ ”’」や三連二重引用符「””” “””」で記述します。
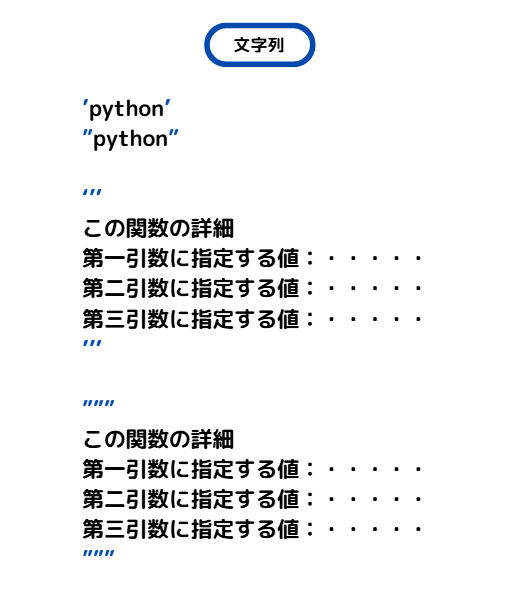
文字列の様々な作成方法
文字列を作成する場合、「’ ‘」シングルクォーテーションなどを利用します。このとき、利用するクォーテーションの種類により、その中の記述内容に違いがでることがあります。
「’ ‘」や「” “」を利用すると一行の文字列を記述できます。
「”’ ”’」や「””” “””」を利用すると複数行の文字列を記述できます。
サンプルプログラム
str1 = 'python is a programming language.'
str2 = "python is a programming language."
str3 = '''
python is a programming language with the following characteristics
Concise and readable syntax
Object-oriented
Interpreted language
Cross-platform and OS-independent
Used for a wide variety of purposes
Can be executed interactively in an interactive shell
Has community and support
'''
str4 = """
python is a programming language with the following characteristics
Concise and readable syntax
Object-oriented
Interpreted language
Cross-platform and OS-independent
Used for a wide variety of purposes
Can be executed interactively in an interactive shell
Has community and support
"""
print(str1)
print(str2)
print(str3)
print(str4)
実行結果
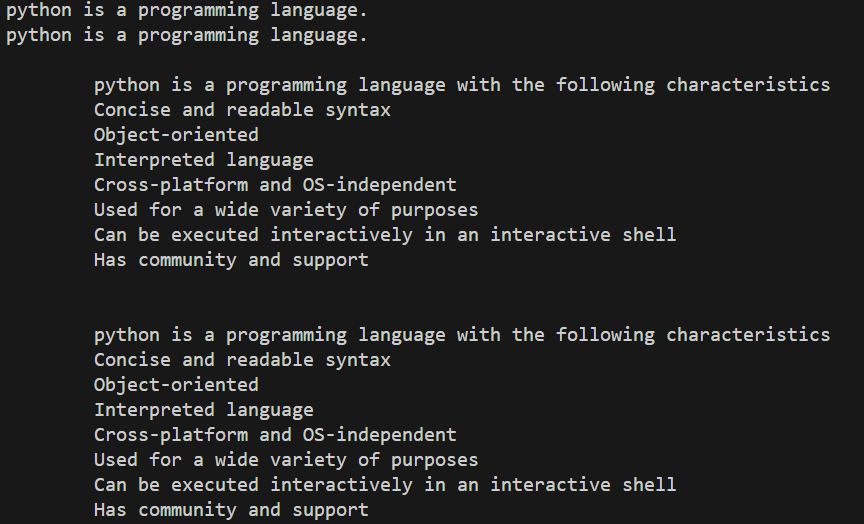
「クォーテーション」を入れ子にする場合
「’ ‘」で表記した文字列では「” “」を文字列の要素として利用できます。
「” “」で表記した文字列では「’ ‘」を文字列の要素として利用できます。
「”’ ”’」や「””” “””」を利用すると、「’ ‘」や「” “」を文字列の要素として利用できます。
サンプルプログラム
str1 = 'python is "a programming language".'
str2 = "python is 'a programming language'."
str3 = '''
python is 'a programming language' with the following characteristics
"Concise and readable syntax"
"Object-oriented"
"Interpreted language"
"Cross-platform and OS-independent"
"Used for a wide variety of purposes"
"Can be executed interactively in an interactive shell"
"Has community and support"
'''
str4 = """
python is "a programming language" with the following characteristics
'Concise and readable syntax'
'Object-oriented'
'Interpreted language'
'Cross-platform and OS-independent'
'Used for a wide variety of purposes'
'Can be executed interactively in an interactive shell'
'Has community and support'
"""
print(str1)
print(str2)
print(str3)
print(str4)
実行結果
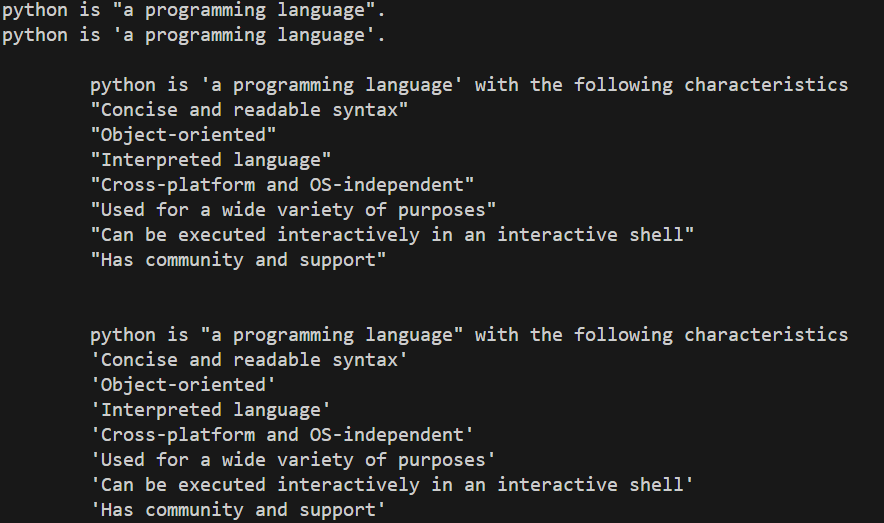
同じ「クォーテーション」を入れ子にする場合
「’ ‘」で表記した文字列では、エスケープシーケンス(特殊文字)の「¥’ ¥’」を文字列の要素として利用できます。
「” “」で表記した文字列では、エスケープシーケンス(特殊文字)の「¥” ¥”」を文字列の要素として利用できます。
「”’ ”’」や「””” “””」を利用する場合は、エスケープシーケンス(特殊文字)での入力を行わなくても文字として利用が可能です。(エスケープシーケンス(特殊文字)を利用することも可能)
サンプルプログラム
str1 = 'python is \'a programming language\'.'
str2 = "python is \"a programming language\"."
str3 = '''
python is \'a programming language\' with the following characteristics
"Concise and readable syntax"
"Object-oriented"
"Interpreted language"
"Cross-platform and OS-independent"
"Used for a wide variety of purposes"
"Can be executed interactively in an interactive shell"
"Has community and support"
'''
str4 = """
python is \"a programming language\" with the following characteristics
'Concise and readable syntax'
'Object-oriented'
'Interpreted language'
'Cross-platform and OS-independent'
'Used for a wide variety of purposes'
'Can be executed interactively in an interactive shell'
'Has community and support'
"""
print(str1)
print(str2)
print(str3)
print(str4)
実行結果
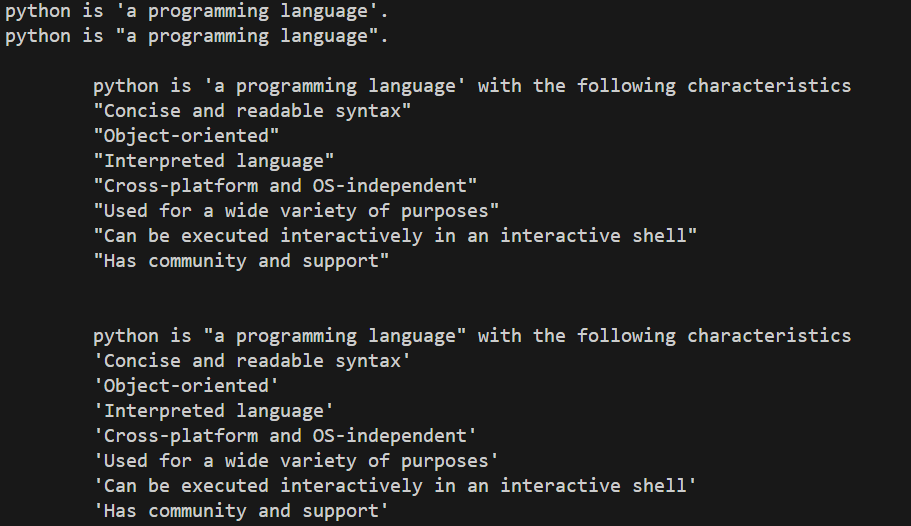
エスケープシーケンス(特殊文字)の利用
エスケープシーケンスは「キーボード上にはないけれど、文章を構成するのに必要な文字」を規定した特殊文字のことです。エスケープシーケンスは通常の文字を「バックスラッシュ」と合わせて利用します。
サンプルプログラム
#ノーマルな文字列です。
str_normal = 'normal normal normal'
#「\t」で「タブ」を表現できます。
str_tab = 'tab \ttab \ttab'
#「\n」で「改行」を表現できます。
str_new_line = 'new line \nnew line \nnew line'
#「\'」で「シングルクォーテーション」を表現できます。
str_single_quote = 'a \'shingle\' quote'
#「\"」で「ダブルクォーテーション」を表現できます。
str_double_quote = "a \"double\" quote"
print(str_normal)
print(str_tab)
print(str_new_line)
print(str_single_quote)
print(str_double_quote)
実行結果
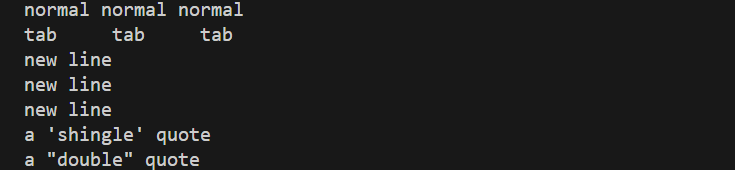
その他の注意点
「文字列」は「’ ‘」シングルクォーテーションや「” “」ダブルクォーテーションを利用して記述します。例えば、「3」のような整数や「7.4501」のような浮動小数点数(または実数)でも「’ ‘」シングルクォーテーションや「” “」ダブルクォーテーションで囲むと、文字列リテラルとなります。
サンプルプログラム
str_integer = '3'
str_float = '7.4501'
print(str_integer)
print(str_float)
実行結果

このように記述した数値は文字列の為、計算には利用できません。
str_integer = '3'
str_float = '7.4501'
print(str_integer + 5)
print(str_float - 2)
実行結果

計算に利用するためにはキャスト(型変換)を行う必要があります。
str_integer = '3'
str_float = '7.4501'
print(int(str_integer) + 5)
print(float(str_float) - 2)
実行結果

今回は以上になります。
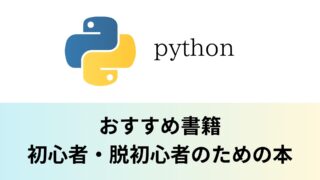
ブックマークのすすめ
「ほわほわぶろぐ」を常に検索するのが面倒だという方はブックマークをお勧めします。ブックマークの設定は別記事にて掲載しています。

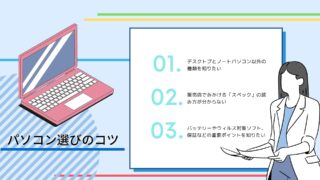