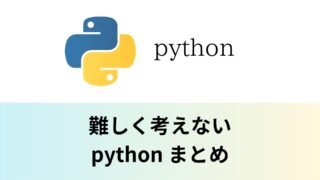
Objective
To understand “built-in functions”.
To understand how to use “id()” of “built-in functions”.
To understand how to use “type()” of “built-in functions”.
Programming languages come standard with several built-in functions. In “python,” about 70 built-in functions are provided to support development using “python”.
built-in functions
Overview of “Built-in Functions” in “Python”
What are built-in functions?
A “built-in function” is a classification of functions, and refers to the functions that come standard with a programming language. On the other hand, a “user-defined function” is a function that is originally created by the programmer when writing a program.
Both functions can be viewed as a single process stored in a single location.
Therefore, This is often likened to a “program components”.
As of version 3.13.1, there are about 71 built-in functions in Python, and programmers can check how to use them in the Python 3.13.1 documentation.(https://docs.python.org/ja/3/library/functions.html)
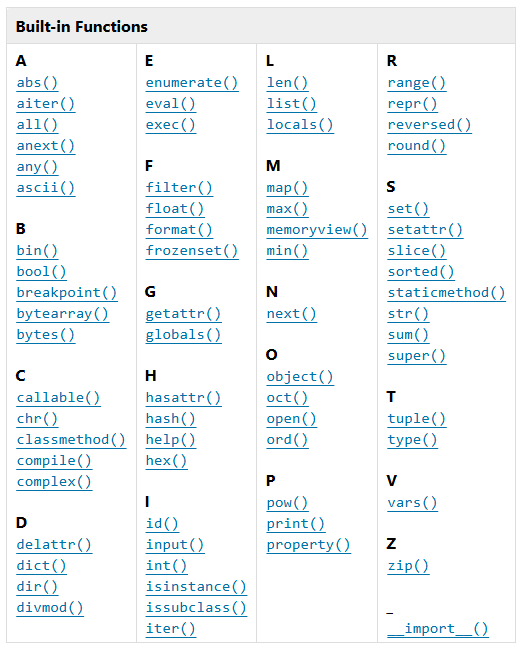
How to use “id()” in “Built-in Functions”
“id(object)” returns the ‘identification value’ of the object specified in the argument. By using “id()”, for example, you can check the object to which a variable is attached.
Click here for a reference of the built-in function “id()”.
When setting a literal as an argument
print('----------------------------------')
print(id(1)) #Directly set an integer literal
print('----------------------------------')
print(id(3.14)) #Directly set a floating-point literal
print('----------------------------------')
print(id('abcdefg')) #Directly set a string literal
print('----------------------------------')
print(id(True)) #Directly set built-in constants
print('----------------------------------')
print(id([1,2,3,4,5])) #Directly set a list
print('----------------------------------')
print(id((1,2,3,4,5))) #Directly set a tuple
print('----------------------------------')
Execution Result( The identification value is returned.)
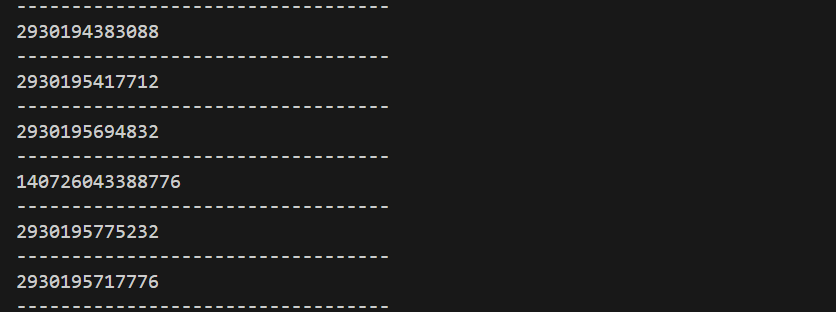
When setting a variable as an argument
int_object = 1
float_object = 3.14
str_object = 'abcdefg'
boolean_object = True
list_object = [1,2,3,4,5]
tuple_object =(1,2,3,4,5)
print('----------------------------------')
print(id(int_object)) #Set an integer literal using a variable.
print(id(1)) #Directly set an integer literal.
print('----------------------------------')
print(id(float_object)) #Set a Floating-point literal using a variable.
print(id(3.14)) #Directly set a floating-point literal.
print('----------------------------------')
print(id(str_object)) #Set a String literal using a variable.
print(id('abcdefg')) #Directly set a string literal.
print('----------------------------------')
print(id(boolean_object)) #Set Built-in Constants using a variable.
print(id(True)) #Directly set built-in constants.
print('----------------------------------')
print(id(list_object)) #Set a list using a variable.
print(id([1,2,3,4,5])) #Directly set a list.
print('----------------------------------')
print(id(tuple_object)) #Set a tuple using a variable.
print(id((1,2,3,4,5))) #Directly set a tuple.
print('----------------------------------')
Execution Result( The identification value is returned.)
The identification value is returned. The case where a variable is specified as an argument and the case where the same value is specified as an argument are lined up to check the difference in the identification value. The same value is returned in all cases except for the list.
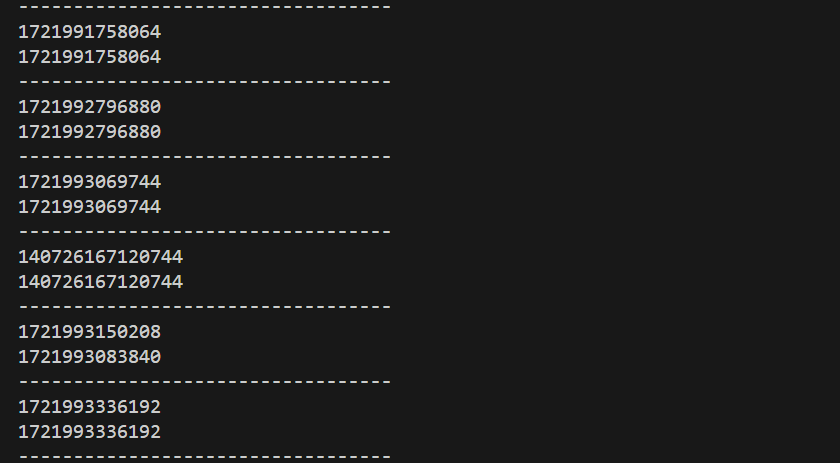
“id()” can check the ‘identification value’ of the object specified in the argument! This is all.
How to use “type()” in “Built-in Functions”
There are two ways to use “type()”. The first is to specify an object by using a single argument. In this case, “type()” returns the type of the object. The second is to use three arguments, which allows class inheritance.
Click here for a reference of the built-in function “type()”.
When only one argument is used (set literal)
“type(object)” returns the ‘type’ of the object specified by the argument.
print('----------------------------------')
print(type(1)) #Directly set an integer literal
print('----------------------------------')
print(type(3.14)) #Directly set a floating-point literal
print('----------------------------------')
print(type('abcdefg')) #Directly set a string literal
print('----------------------------------')
print(type(True)) #Directly set built-in constants
print('----------------------------------')
print(type([1,2,3,4,5])) #Directly set a list
print('----------------------------------')
print(type((1,2,3,4,5))) #Directly set a tuple
print('----------------------------------')
Execution Result(Each type is returned.)
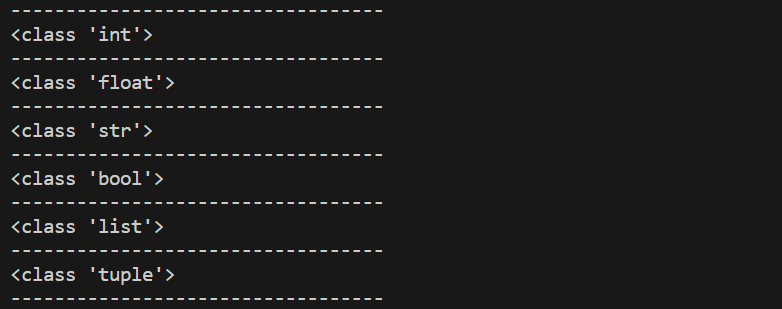
When only one argument is used (set variable)
The “type()” can be used to check the “type” of the object to which the variable is attached.
int_object = 1
float_object = 3.14
str_object = 'abcdefg'
boolean_object = True
list_object = [1,2,3,4,5]
tuple_object =(1,2,3,4,5)
print('----------------------------------')
print(type(int_object)) #Set an integer literal using a variable.
print(type(1)) #Directly set an integer literal.
print('----------------------------------')
print(type(float_object)) #Set a Floating-point literal using a variable.
print(type(3.14)) #Directly set a floating-point literal.
print('----------------------------------')
print(type(str_object)) #Set a String literal using a variable.
print(type('abcdefg')) #Directly set a string literal.
print('----------------------------------')
print(type(boolean_object)) #Set Built-in Constants using a variable.
print(type(True)) #Directly set built-in constants.
print('----------------------------------')
print(type(list_object)) #Set a list using a variable.
print(type([1,2,3,4,5])) #Directly set a list.
print('----------------------------------')
print(type(tuple_object)) #Set a tuple using a variable.
print(type((1,2,3,4,5))) #Directly set a tuple.
print('----------------------------------')
Execution Result(Each type is returned.)
The literal type that is tied to the variable is returned even if the variable is used.
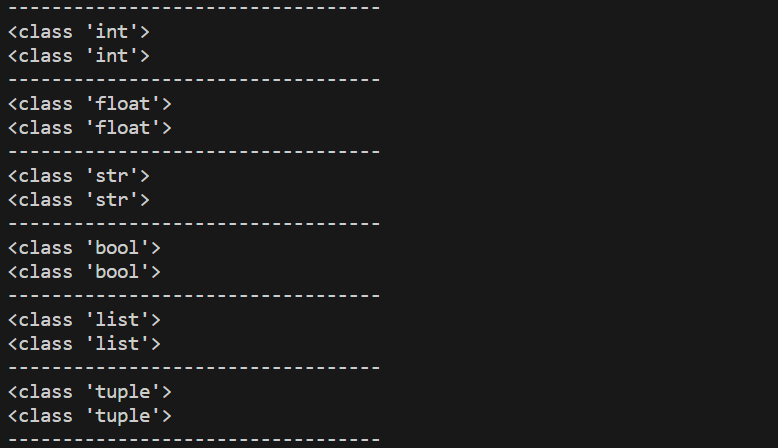
When three arguments are used(Class Inheritance)
“type(name, bases, dict, kwds)” creates a derived class with the name specified in the first argument ‘name’ by inheriting the class specified in the second argument ‘bases’. In the third argument, attributes and methods in the class are copied to “dict” as dictionary types, and these can be specified and modified (kwds).
type(derived class name, base class, dict, **kwds (attributes and methods))”」
# ---base class---
class Car:
gas = 100
water = 100
battery = 100
speed = 0
acceleration = 10
def accelerator(self):
while self.speed <= 80:
if self.speed == 80:
break
else:
self.speed += self.acceleration
print('The speed is {}.'.format(self.speed))
return self.speed
def brake(self):
while self.speed >= 0:
if self.speed == 0:
break
else:
self.speed -= self.acceleration
print('The speed is {}.'.format(self.speed))
return self.speed
# ---Inherited Classes---
class TOYOTA(Car):
acceleration = 20
# ---Using Inherited Classes---
carolla = TOYOTA()
carolla.accelerator()
carolla.brake()
Execution Result
The speed increases by 20 to 80, then decreases by 20 to 0.
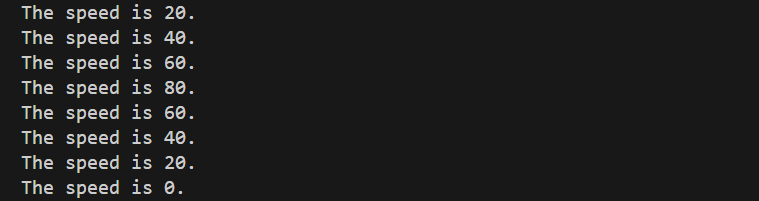
# ---base class---
class Car:
gas = 100
water = 100
battery = 100
speed = 0
acceleration = 10
def accelerator(self):
while self.speed <= 80:
if self.speed == 80:
break
else:
self.speed += self.acceleration
print('The speed is {}.'.format(self.speed))
return self.speed
def brake(self):
while self.speed >= 0:
if self.speed == 0:
break
else:
self.speed -= self.acceleration
print('The speed is {}.'.format(self.speed))
return self.speed
# ---Inherited Classes---
TOYOTA = type('TOYOTA', (Car,), dict(acceleration = 20))
# ---Using Inherited Classes---
carolla = TOYOTA()
carolla.accelerator()
carolla.brake()
Execution Result
The same results are obtained as before.(The speed increases by 20 to 80, then decreases by 20 to 0.)
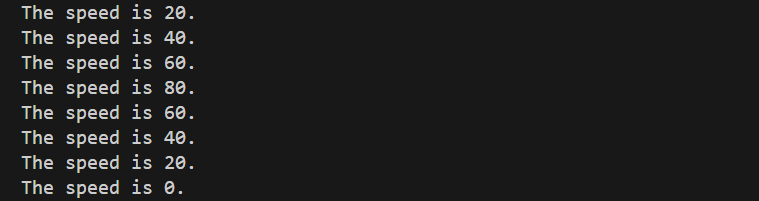
The most common use of “type()” is to check the type, but it is recommended to know that there is also a pattern of using three arguments since you may see it in some modules or frameworks.
That’s all for this time.
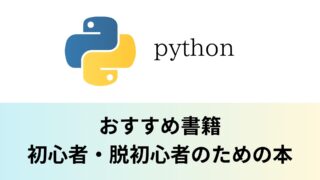
ブックマークのすすめ
「ほわほわぶろぐ」を常に検索するのが面倒だという方はブックマークをお勧めします。ブックマークの設定は別記事にて掲載しています。

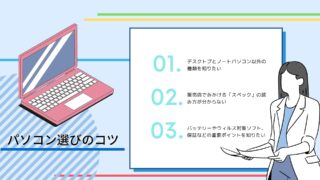