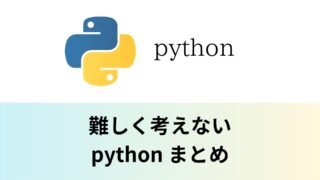
python| まとめ | 現役エンジニア&プログラミングスクール講師「python」のまとめページです。pythonに関して抑えておきたい知識や文法やにについて記事をまとめています。まとめページの下部には「おすすめの学習書籍」「おすすめのITスクール情報」「おすすめ求人サイト」について情報を掲載中...
目次
目標
- 商品編集時に以前の商品名や価格をテキストボックスへ表示させる
- アプリケーション内の画像を選択できるようにする
- 商品に「販売中」「完売」「在庫切れ」「販売中止」ステータスを作成して「完売」「在庫切れ」は選択不可とし、「販売中止」は表示しない
商品管理アプリケーションの修正
商品管理アプリケーション修正
ここからは、これまで、商品管理アプリケーションで不便と感じていた部分について修正を行います。具体的には次の通りです。
- 商品一覧の編集ボタンをクリックしたときに、表示される編集画面で、編集前の商品名、価格、カテゴリーを初期値でセットさせます。
(Git では release-products-001 ブランチで修正) - 商品編集で画像をアップロードする以外に、既にアップロード済みの画像からも画像を選択できるようにします。
(Git では release-products-002 ブランチで修正) - 商品に「販売中」「完売」「在庫切れ」「販売中止」ステータスを作成して「完売」「在庫切れ」は選択不可とし、「販売中止」は表示しないようにします。
(Git では release-products-003 ブランチで修正)
release-productsブランチの作成
release-productsブランチの作成
商品管理アプリケーションは既に基本となる構成については作成を完了しています。このように、機能の追加漏れがあるような場合は、再度、featureブランチを作成するよりも、releaseブランチとしてブランチを作成して作業を行うのが一般的です。
Git Bushを立ち上げるとプロンプトの右側に(develop)と表示され、現在のブランチがdevelopブランチであることが分かります。(次の表示はVisual Studio CodeのGit Bushのプロンプトです。)
User@dellDevice MINGW64 ~/Desktop/FlaskProj (develop)
次のようにコマンドを入力していきます。次のように表示されるはずです。
$ git status

$ git checkout -b release-products-003

$ git status

商品管理アプリケーションの修正(release-products-003)
app.pyの編集(productsapp内)
app.pyファイルの「edit_product関数」を次のように編集します。
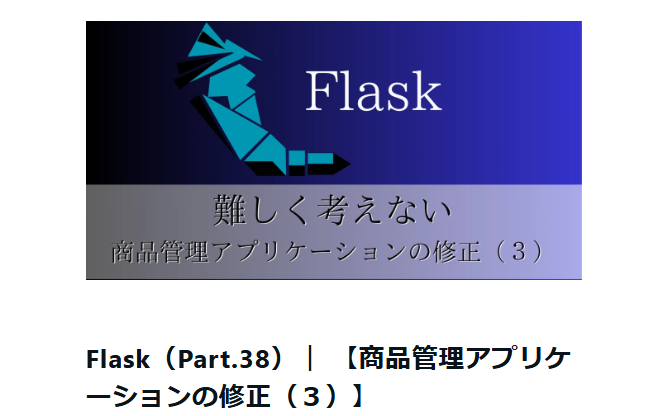
今回は以上になります。
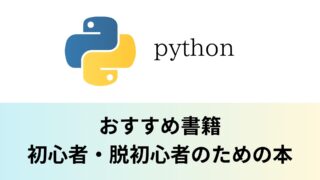
「python」おすすめ書籍 ベスト3 | 現役エンジニア&プログラミングスクール講師「python」の学習でお勧めしたい書籍をご紹介しています。お勧めする理由としては、考え方、イメージなどを適切に捉えていること、「生のpython」に焦点をあてて解説をしている書籍であることなどが理由です。勿論、この他にも良い書籍はありますが、特に質の高かったものを選んで記事にしています。ページの下部には「おすすめのITスクール情報」「おすすめ求人サイト」について情報を掲載中。...
ブックマークのすすめ
「ほわほわぶろぐ」を常に検索するのが面倒だという方はブックマークをお勧めします。ブックマークの設定は別記事にて掲載しています。

「お気に入り」の登録・削除方法【Google Chrome / Microsoft Edge】「お気に入り」の登録・削除方法【Google Chrome / Microsoft Edge】について解説している記事です。削除方法も掲載しています。...
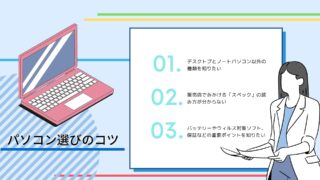
【パソコン選び】失敗しないための重要ポイント | 現役エンジニア&プログラミングスクール講師【パソコン選び】失敗しないための重要ポイントについての記事です。パソコンのタイプと購入時に検討すべき点・家電量販店で見かけるCPUの見方・購入者が必要とするメモリ容量・HDDとSSDについて・ディスプレイの種類・バッテリーの持ち時間や保証・Officeソフト・ウィルス対策ソフトについて書いています。...
Blueprint Flask Flask-SQLAlchemy Jinja2 MVT python session SQLite アップロード エンジニア セッション テンプレートエンジン バリデーション フレームワーク ルーティング 作成方法 初心者 利用方法 注意点 画像 統合
この続きはNoteとなります。