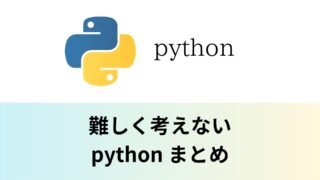
目標
- 全てのテーブル状況を確認できるテンプレートを実装する(日時での抽出)
- 各テーブルの注文状況を確認できるテンプレートを実装する
レジ管理アプリケーションの作成
レジ管理アプリケーションの作成
ここからは、商店スタッフが利用するためのレジ管理アプリケーションを作成していきます。具体的には、全てのテーブル状況を確認できたり、各テーブルの注文状況を確認でき、ユーザー側では行えない注文済みの商品の取り消しや、会計を完了させる機能を実装します。
feature-registerappブランチの作成
Git Bushを立ち上げdevelopブランチから次のコマンドを入力してfeature-registerappブランチを作成して、ブランチの切り替えを行います。
git checkout -b feature-registerappコマンド

プロンプトに(feature-registerapp)と表示されます。レジ管理アプリケーションはこのブランチで作成していきます。
レジ管理アプリケーションに必要なディレクトリとファイルの作成
Visual Studio Codeのエクスプローラーから appsディレクトリ に registerappディレクトリを作成します。
apps/registerappディレクトリに次のディレクトリとファイルを作成します。※作成するファイルについては、名前や中身について、今後変更することものもあります。
- static/css/style.css
- templates/register_base.html
- tamplates/register_orders_status.html
- tamplates/register_bill.html
- tamplates/register_list.html
- app.py
- forms.py
- models.py
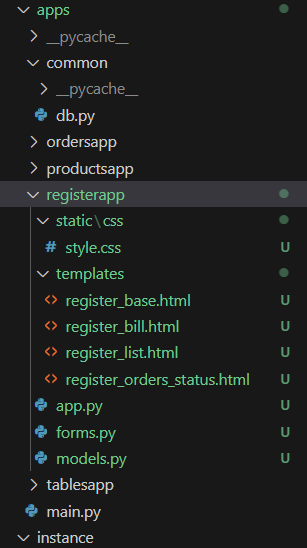
レジ管理アプリケーションのプログラミング
この記事では、全てのテーブル状況(伝票)を確認できるテンプレートとロジックを実装します。その時に、ある日時以降の伝票のみを出力できるようにします。この為、伝票の作成時間(created_at)をBillモデルに追加します。
tzdata のインストール
Python の zoneinfo モジュールを使用して”Asia/Tokyo” などの特定のタイムゾーン情報を扱う場合、tzdata が必要となります。
「pip install tzdata」コマンドの利用
(venv) C:\Users\User\Desktop\FlaskProj>pip install tzdata
と入力します。

models.pyの編集(ordersapp内)
ordersapp内のmodels.pyファイルを次のように編集します。
from datetime import timedelta, timezone
from sqlalchemy.sql import func
from apps.common.db import db
JST = timezone(timedelta(hours=9))
# 伝票(Bill)モデル
class Bill(db.Model):
id = db.Column(db.Integer, primary_key=True)
status = db.Column(
db.String(20), default="paid"
) # 伝票のステータス(Order in progress, pending, paid)
table_id = db.Column(
db.Integer, db.ForeignKey("table.id"), nullable=False
) # テーブルとの関連
orders = db.relationship("Order", backref="bill", lazy=True, cascade="all, delete")
table = db.relationship(
"Table", backref="bill_table", uselist=False, overlaps="table,table"
) # 1つのテーブルに1つの伝票
created_at = db.Column(db.DateTime, default=func.now(), server_default=func.now())
def __repr__(self):
return f"<Bill {self.id} - Status: {self.status}>"
def get_created_at_jst(self):
"""JST に変換して取得"""
if self.created_at is None:
return None
return self.created_at.replace(tzinfo=timezone.utc).astimezone(JST)
# 注文(Order)モデル
class Order(db.Model):
id = db.Column(db.Integer, primary_key=True)
table_id = db.Column(db.Integer, db.ForeignKey("table.id"), nullable=False)
bill_id = db.Column(db.Integer, db.ForeignKey("bill.id"), nullable=True)
product_id = db.Column(db.Integer, db.ForeignKey("product.id"), nullable=False)
product_name = db.Column(db.String(100), nullable=False)
quantity = db.Column(db.Integer, nullable=False)
total = db.Column(db.Float, nullable=False) # Float に変更
table = db.relationship("Table", backref="orders", lazy=True)
product = db.relationship("Product", backref="orders", lazy=True)
def __repr__(self):
return f"<Order {self.id} - Table {self.table_id} - {self.product_name} x {self.quantity}>"
created_at = db.Column(db.DateTime, default=func.now(), server_default=func.now())
SQLAlchemy を使ったデータベースのカラム定義しています。
db.DateTime:日時型のカラムです。
server_default=func.now(): データベース側で、レコードが作成された時点の CURRENT_TIMESTAMP(現在時刻)を設定しています。
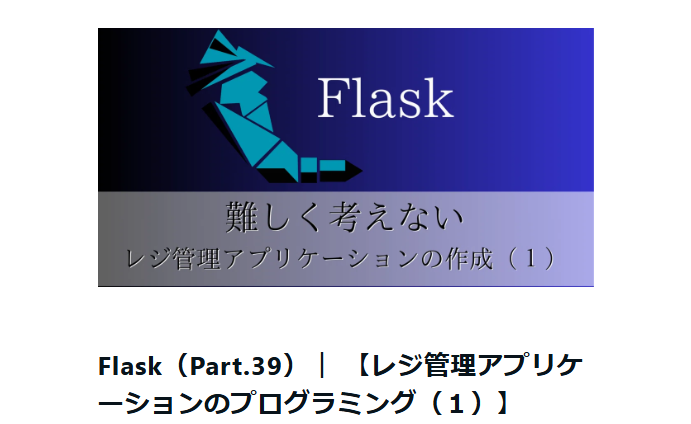
今回は以上になります。
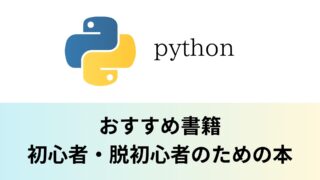
ブックマークのすすめ
「ほわほわぶろぐ」を常に検索するのが面倒だという方はブックマークをお勧めします。ブックマークの設定は別記事にて掲載しています。

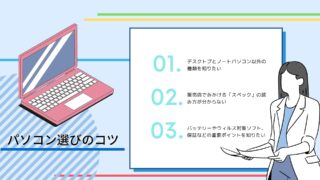
この続きはNoteとなります。