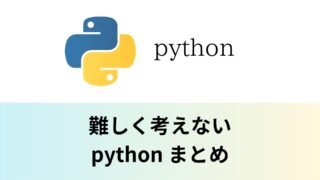
Objective
To understand “variables.”
This article deals with “variables” as a foundation of python. In learning programming, “variables” are often compared to boxes, but in this article we will think of them as “labeling” or “ linking” to “literals”. Also, learning about “variables” requires knowledge of “literals” and “assignment statements.
Python Basics (Variables)
In learning programming, “variables” are often compared to “boxes”, but in this article we will learn to compare them to “labeling” or “linking”. This “labeling” can be said to be the storage of “IDs” for “literals” (immutable values).
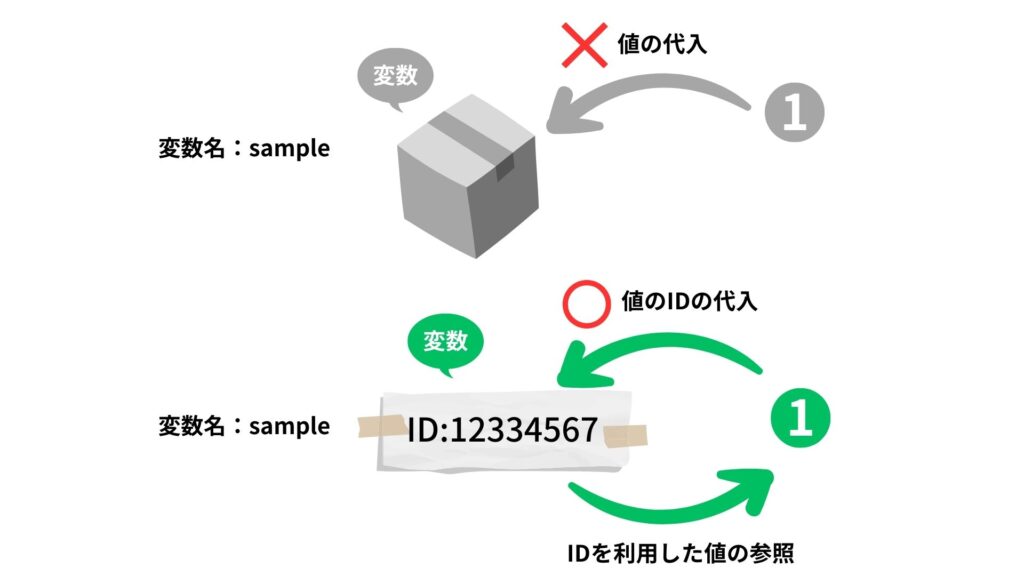
【English translation of captcha】
変数 → Variables
値の代入 → Value Assignment
値のIDの代入 → Assigning an ID to a value
IDを利用した値の参照 → Referencing a value by its ID
The metaphor aside, in any case, in programming, the use of variables makes it possible to reuse values. Without variables, the results of executing a program cannot be reused.
Take the following program as an example. If you do not use variables, you will need to enter the necessary values each time.

In contrast, the use of “variables” makes it possible to reuse values.
The capture below shows the assignment of a “reference to a string” to a variable named “alp” using an assignment statement. In “alp = ‘abcd’”, the reference to ‘abcd’ is assigned. In “alp += ‘efgh’”, ‘abcd’ + ‘efgh’ is processed and finally the reference to ‘abcdefgh’ is reassigned.
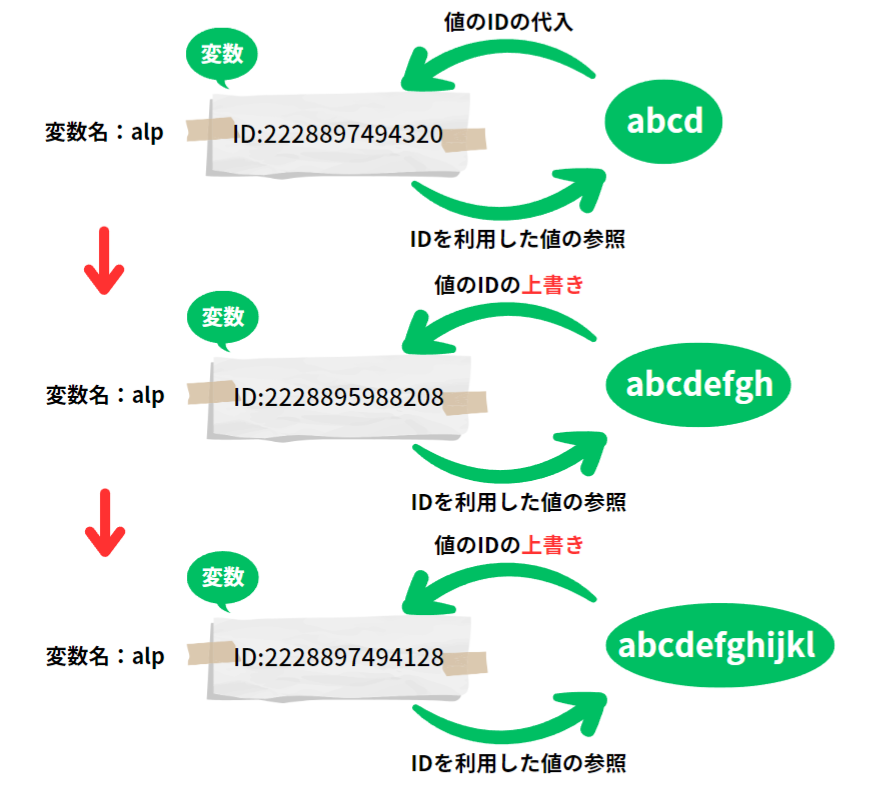
【English translation of captcha】
変数名 → Variable name
IDを利用した値の参照 → Referencing a value by its ID
値のIDの上書き → Overwriting the ID of a value
Static and Dynamic typing
Variables in “python” are “dynamic typing”. There are some of programming languages use “static typing,” in which not only literals but also variables themselves are assigned a type. This function specifies what literals can be used in the variable itself and how much space is available for each literal.
In python, variables do not have types. It is literals that have types, and variables merely store references to literals.
「Static typing」
Static typing” specifies the ‘type’ to be used for a ”variable. This allows the “variable” to set if it can use which types and how much space it needs, which can improve performance.
In addition, Wikipedia describes it as follows
静的型付け(せいてきかたづけ、英: static typing)は、値やオブジェクトの型安全性を、コンパイル時に検証するというコンピュータプログラミングの型システムの方法である。型の検査はソースコードの解析によって行われる。変数代入、変数束縛、関数適用、型変換といったプログラム記述箇所での型安全性がチェックされる。型エラーの場合は、コンパイルエラーに繋げられることが多い。
English translation
Static typing is a method of computer programming type systems in which the type safety of a value or object is verified at compile time. Type checking is done by source code analysis. Type safety is checked at program description points such as variable assignment, variable binding, function application, and type conversion. Type errors often lead to compile-time errors.
https://ja.wikipedia.org/wiki/%E9%9D%99%E7%9A%84%E5%9E%8B%E4%BB%98%E3%81%91
For example, in “C #”, the type “int” is specified before the variables “num1” and “num2”. In addition, the type is also specified as a temporary argument or return value for the method (int SaySomthing(int input1, int input2){}).
The return type, i.e., the type of the value returned by the method, is the “int” preceding the method name “SaySomthing. Thus, “static typing” specifies the type of the value returned by a “variable” or “method (function).
using System;
namespace Sample
{
class Sample
{
static int SaySomething(int input1, int input2){
return (input1 + input2);
}
static void Main(string[] agrs)
{
int num1 = 1234;
int num2 = 4321;
Console.WriteLine("静的型付けを利用しています。" + SaySomething(num1, num2));
}
}
}
「Dynamic typing」
Dynamic typing does not specify a type for a variable. This allows variables to refer to values in a flexible manner.
In addition, Wikipedia describes it as follows
動的型付け(どうてきかたづけ、英: Dynamic typing)とは、値やオブジェクトの型安全性を、実行時に検証するというコンピュータプログラミングの型システムの方法である。型の検査は実行時のプロセス上で行われて、ランタイムシステムの実行時型情報(RTTI)が照会されるなどして解析される。
動的な型チェックは、代入、束縛、関数適用、ダウンキャスト、ディスパッチ、バインディングといった所で行われる。なお、コンパイル時やインタプリタ開始時の最適化によってすでに型安全性が保証されている所は省略される。動的型付けの言語では、引数や返り値や変数宣言への型注釈が省略されやすくなる。
English translation
Dynamic typing is a type system method of computer programming in which the type safety of a value or object is verified at runtime. Type checking is performed on the process at runtime and analyzed, for example, by querying the runtime system’s runtime type information (RTTI).
Dynamic type checking is performed at assignment, binding, function application, downcasting, dispatching, and binding. Note that type safety is omitted where type safety is already guaranteed by compile-time or interpreter start-up optimizations. In dynamically typed languages, type annotations on arguments, return values, and variable declarations are more likely to be omitted.
https://ja.wikipedia.org/wiki/%E5%8B%95%E7%9A%84%E5%9E%8B%E4%BB%98%E3%81%91
That is all for this issue.
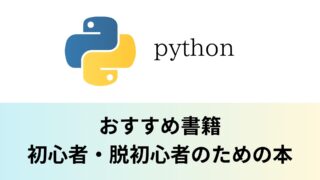
ブックマークのすすめ
「ほわほわぶろぐ」を常に検索するのが面倒だという方はブックマークをお勧めします。ブックマークの設定は別記事にて掲載しています。

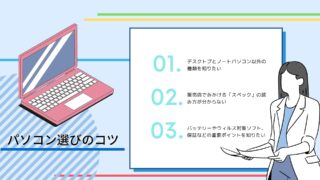