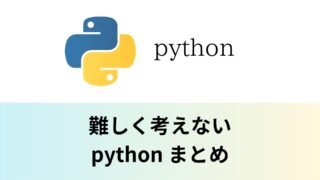
目標(Objectives)
「for-else文」について理解する。
To understand “for-else statements”.
反復構造(for文)
Iterative structure (for statement)
基本制御構造の復習
Review of basic control structures
基本制御構造とは(再掲載)
What is basic control structure (repetition)
「制御構造」のうち、基本とする3つの構造として「順次構造」「選択構造」「反復構造」が存在します。これらは「基本制御構造」と呼ばれて、次の構造をもっています。
- 「順次構造」…プログラムを書かれた順番で実行します。
- 「選択構造」…条件の成立・不成立によって実行するプログラムを選択します。
- 「反復構造」…条件の成立・不成立によって実行するプログラムを反復します。
Among the “control structures,” there are three basic structures: sequential, selective, and repetitive. These are called “basic control structures” and have the following structure.
- Sequential structure: Programs are executed in the order in which they are written.
- Selective structure: The program is selected to be executed according to whether the condition is satisfied or not.
- Iterative structure: The program to be executed is iterated according to whether the condition is satisfied or not.
「for-else文」の記述
Description of “for-else” statement
「for-else文」の文法
Syntax of “for-else statement
pythonの「for文」では「else節」を利用できます。この「else節」の処理は「for文」内の処理が「break文」などで終了されないで、無事全て完了した場合に実行されます。
The for statement in python allows the use of an else clause. This else clause is executed when all processing in the for statement is completed without being terminated by a break statement.
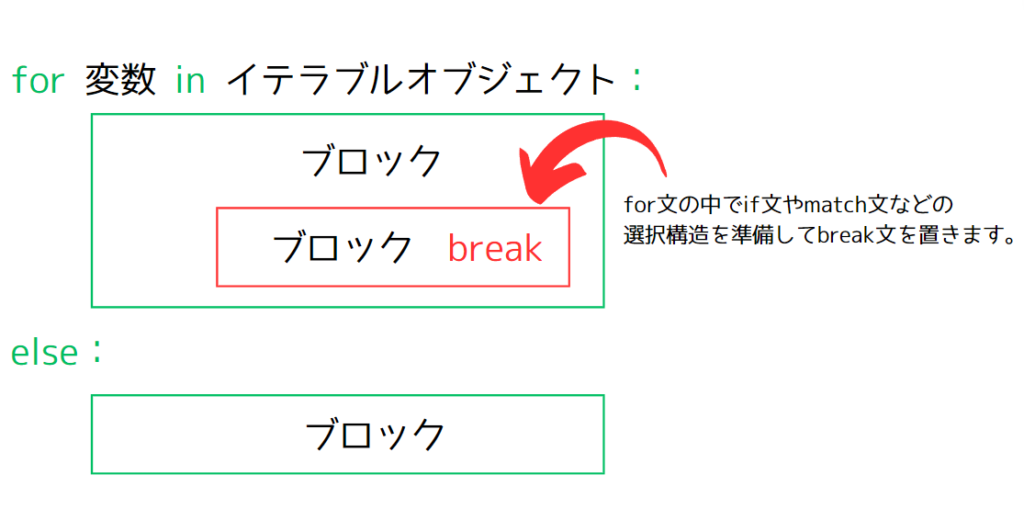
【English translation of captcha】
変数
→The variable
イテラブルオブジェクト
→Iterable objects
ブロック
→Block
for文の中でif文やmatch文などの選択構造を準備してbreak文を置きます。
→Prepare a selection structure such as if and match statements in a for statement and place a break statement.
-320x180.jpg)
例えば下のプログラムの実行結果や動きは次のようになります。
For example, the execution results and movements of the program below are as follows
students_score = [['A','A','A'],['A','B','B'],['B','A','A'],['A','A','C'],['A','C','B']]
students_number = int(input('受講生のIDを「1~5」で入力してください。'))
if students_number >= 1 and students_number <= 5:
for student_score in students_score[students_number - 1]:
if student_score == 'C':
print("C評価があります。追加課題が必要です。")
break
pass
else:
print("Cの評価はありません。")
else:
print("正しくIDを入力してください。")
実行結果(for文の処理が全て行われる場合)
Execution Result (When all processing of the for statement is done)
for文の繰り返しが全て行えた場合は処理がelse節へ移ります。その結果、「Cの評価はありません。」が表示されることになります。
If all iterations of the for statement are done, processing moves to the else clause. As a result, “C is not evaluated. will be displayed.

動作(for文の処理が全て行われる場合)
Operation (when all processing of the for statement is completed)
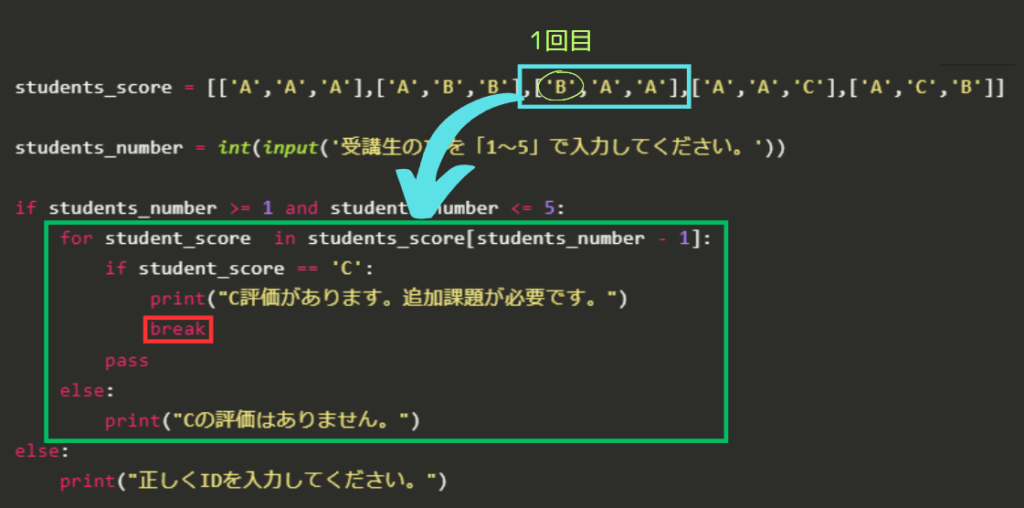
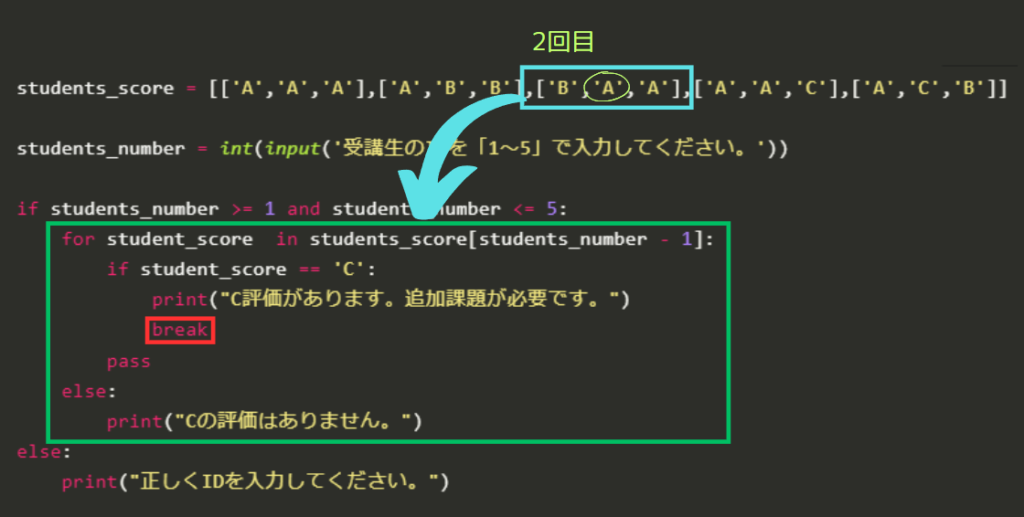
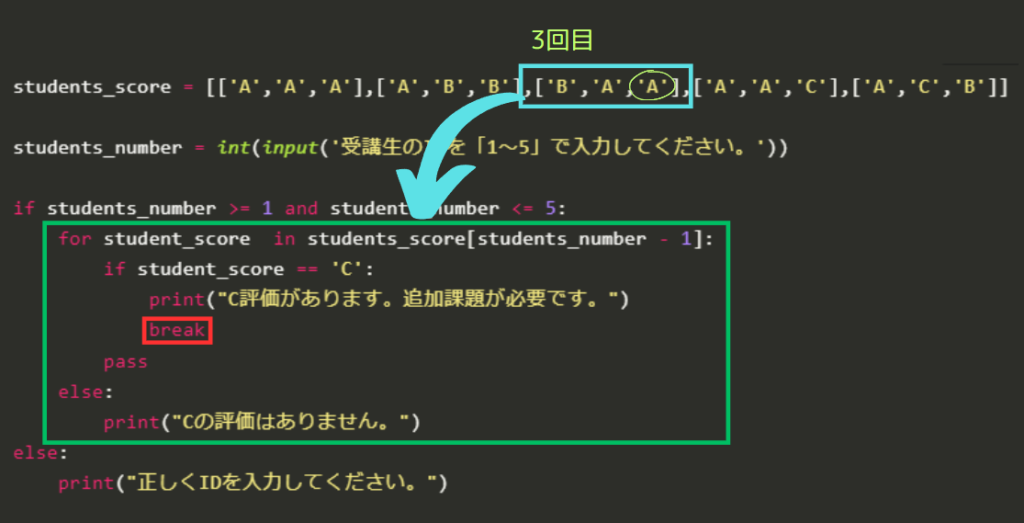
実行結果(for文の処理がbreak文で強制終了する場合)
Execution Result (When the processing of a for statement is forced to terminate with a break statement)
次の場合は「break文」がかかってしまった場合です。for文での処理が全て終わる前に強制終了した場合にはelse節の処理は行われません。
The following is a case where a “break statement” is applied: If the for statement is terminated before all processing in the for statement is completed, the else clause is not processed.

動作(for文の処理がbreak文で強制終了する場合)
Operation (when the processing of a for statement is forced to terminate with a break statement)
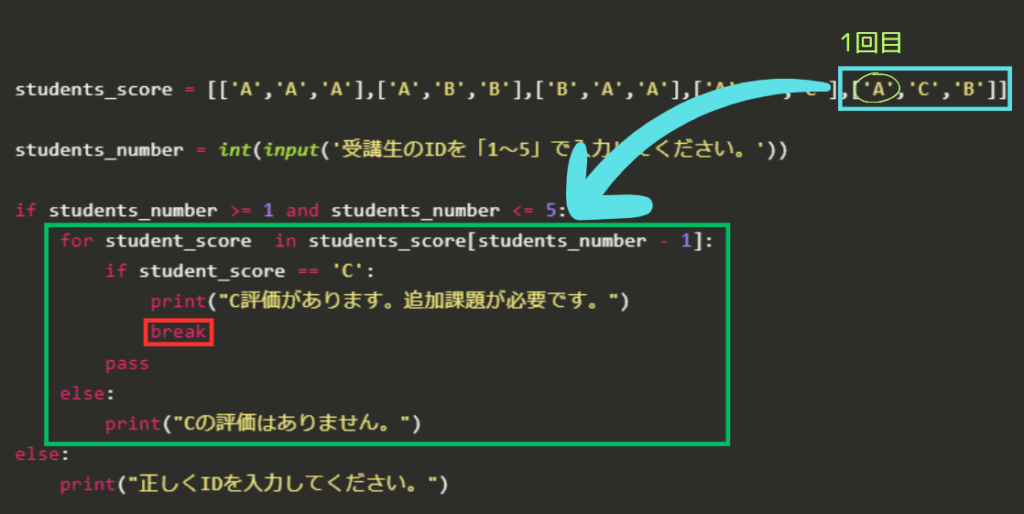
ここで、for文内の「break文」が動きます。これによって、「for-else文」から処理が抜けます。
Here, the “break statement” in the for statement moves. This causes the process to exit from the “for-else” statement.
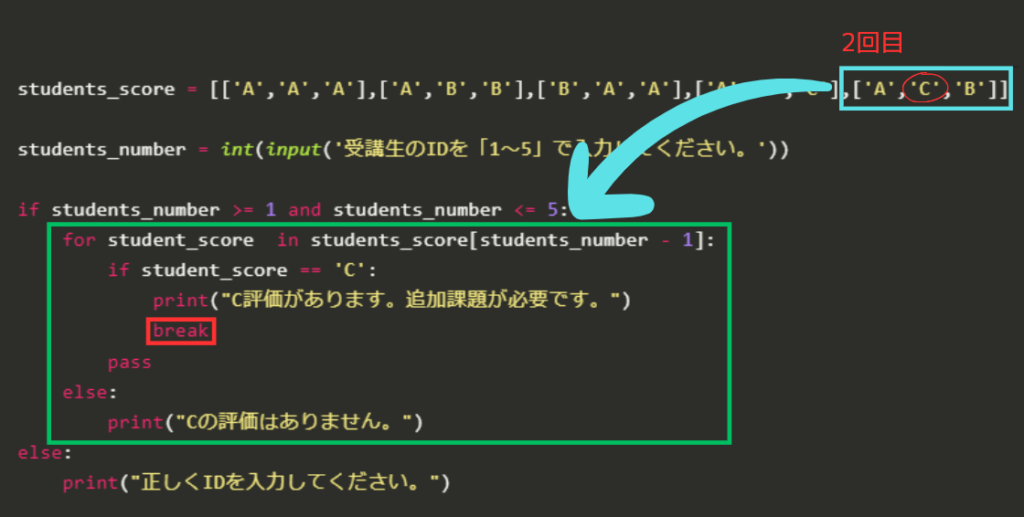
今回は以上になります。
That’s all for this time.
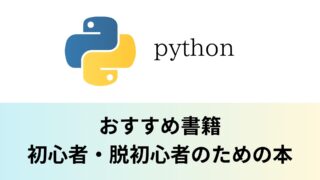
ブックマークのすすめ
「ほわほわぶろぐ」を常に検索するのが面倒だという方はブックマークをお勧めします。ブックマークの設定は別記事にて掲載しています。

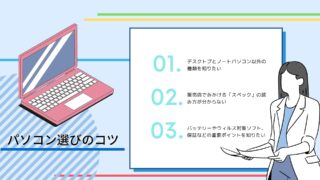