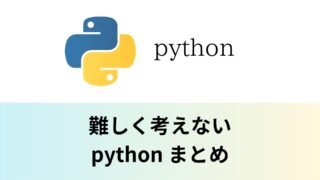
Objectives
Understand ‘operator’ and ‘operand’.
Understand ‘operator and expression precedence’.
Overview of operators
When you think of ‘learning programming’, ‘variables’, ‘control structures’ and ‘classes’ come to mind, but before you learn about them, you need to know about the ‘symbols’ used in programming. Knowing about these ‘symbols’ will make learning programming easier.
‘Operator’ and ‘Operand’
‘Using operators’
Before learning the ‘operators’, enter the following into the ‘interactive shell (python console)’. You will see the calculation results returned.
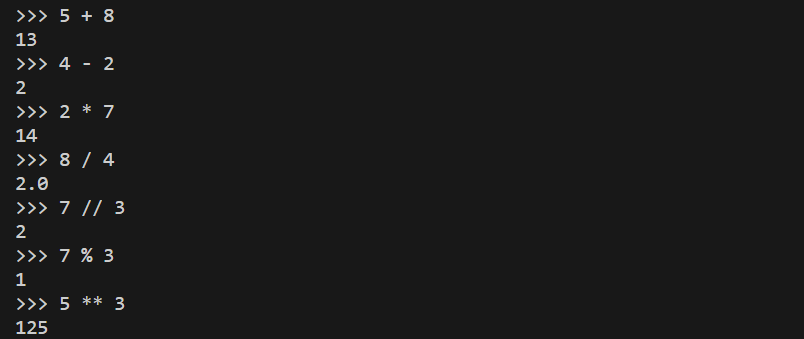
‘Operator’
‘Operator’ is ‘symbols’ used in the four arithmetic operations (addition, subtraction, multiplication, division, etc.), value comparisons and logic to find rules of value. There are the following types of operators.
Operator | Usages |
Arithmetic operators | To perform four arithmetic operations |
Comparison operators | To compare values and return a true or false value |
Logical operators | To derive rules of value |
Concatenation operators | To concatenate strings with strings ※Cannot be used in python to concatenate numbers and strings. |
‘Arithmetic operators’
Arithmetic operators include ‘addition’, ‘subtraction’, ‘multiplication’, ‘division’, ‘remainder’ and ‘power’.
Arithmetic operators | Meaning |
+ | Adds the left and right values. (Addition) |
– | Subtracts the left and right values. (Subtract) |
* | Multiply the left and right values. (Multiply) |
/ | Divide the left value by the right value. (division) |
// | Divide the left value by the right value to obtain the ‘quotient’. (truncated division) |
% | Divide the left value by the right value to obtain the ‘remainder’. (remainder) |
** | Power the left value by the right times (power). |
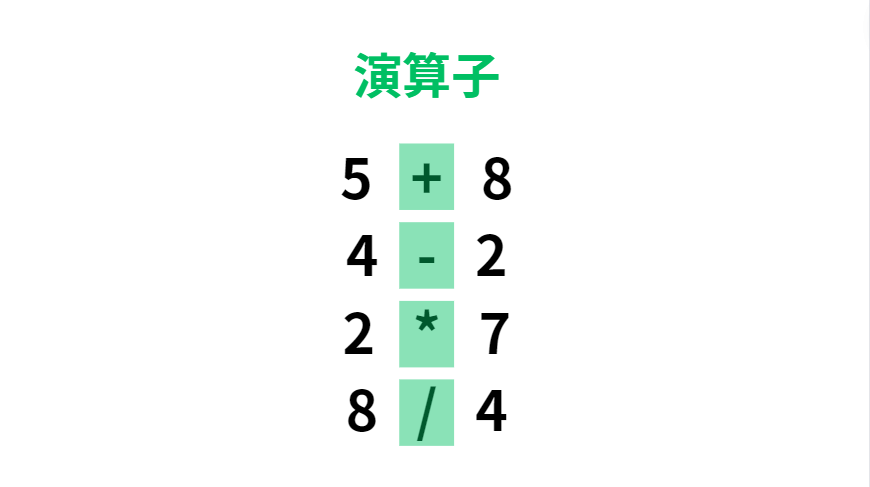
【English translation of captcha】
演算子 → operators
‘Operand’
An ‘operand’ is a ‘value’ that is the object of an operation. The ‘operand’ is the following part
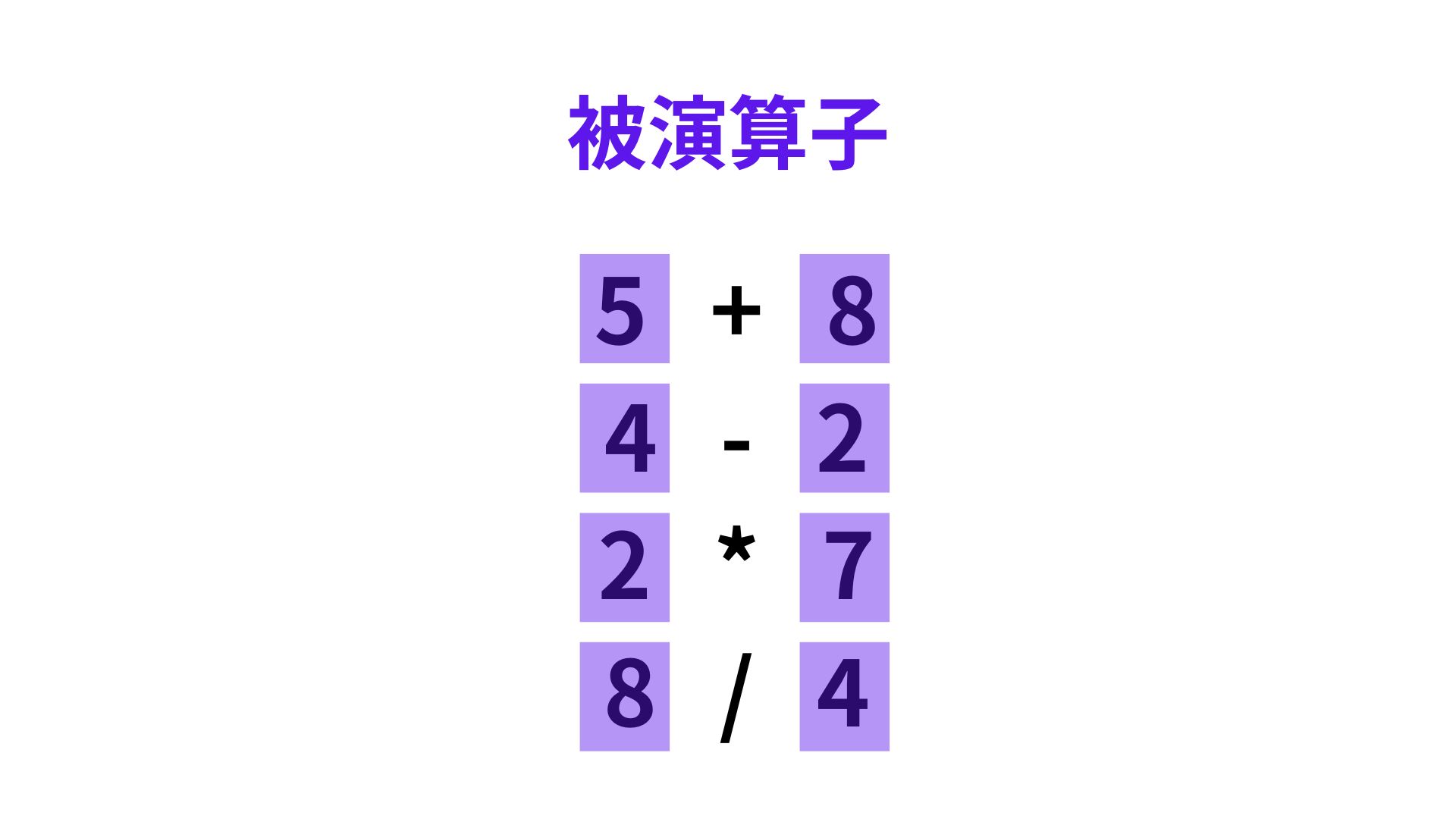
【English translation of captcha】
演算子 → operand
‘Operator and expression precedence’
‘Operators’ have priorities, so that when operators or expressions with different priorities are lined up, the operation with the highest priority is performed first. Commonly used for simple calculations are the ‘1’ expression join, the ‘4’ to ‘7’ arithmetic operators and the ’18’ assignment statement (which is not an operator in the grammar).
Priority | Operators | Meaning |
1 | () 、[]、 {key: value}、{} | Expression binding (tuple notation), list notation, Dictionary notation, set notation |
2 | x[n]、x[n:m]、 x(args *kwargs) x.attr | Indexes, slices, Function calls, Attribute references |
3 | await Expression | await Asynchronous (pause in processing) |
4 | ** | Power |
5 | +x、-x、~x | Positive numbers (unary +), Negative numbers (unary -), Bit inversion |
6 | *、/、//、%、@ | Multiplication, division, Truncated division, Remainder, Matrix multiplication |
7 | +、- | Addition, Subtraction |
8 | <<、>> | Shift operation (shift left, shift right) |
9 | & | Bitwise logical conjunction (AND) |
10 | ^ | Bitwise exclusive or (XOR) |
11 | | | Bitwise OR (OR) |
12 | in、not in、is、is not、 >、>=、<、<=、!=、==、<> | Comparisons (Attributional, Identity and Value comparisons) |
13 | not x | Logical negation (NOT) |
14 | and | Logical conjunction (AND) |
15 | or | OR (logical OR) |
16 | if … elif … else | Condition |
17 | lambda | lambda |
18 | =、:= | Assignment statement ※Not grammatically an operator |
For example, if the following calculation is performed, the order of operations changes as follows.

First, the basic premise is that programmes are read ‘top to bottom’ and ‘left to right’. In the case of the expression below, the programme is a single line, so it is read from left to right.
In order, “answer” is read and “=” is read. At this point, the “=” in the assignment statement is read. Then the next operator to be read, “6”, and the next operator “+” are read, and the priorities of “=” and “+” are compared.
answer = 6 + 7 – 5 * (-4) * (4 + 6 / 3) + 5
As ‘+’ is the operation that takes precedence over ‘=’ from the precedence of the two, it is read further to the next operator.
answer = 6 + 7 – 5 * (-4) * (4 + 6 / 3) + 5
‘+’ and ‘-‘ have the same priority, so 6+7 is processed.
The expression changes to following and read further to the right. Now the priority is compared between ‘-‘ and ‘‘. The ‘‘ has a higher priority, so the calculation moves backwards.
answer = 13 – 5 * (-4) * (4 + 6 / 3) + 5
The bracketed expression is then read. The bracketed expression contains an expression for a negative number. It is then read up to the operator.
answer = 13 – 5 * (-4) * (4 + 6 / 3) + 5
Two asterisks(*) in a row. The priority is of course the same, so only the left-hand asterisk is processed.
answer = 13 – (-20) * (4 + 6 / 3) + 5
Further reading the formula on the right.
answer = 13 – (-20) * (4 + 6 / 3) + 5
()There is a bracketed formula, so the calculations in this take precedence.
answer = 13 – (-20) * (4 + 6 / 3) + 5
The “/” has a higher priority than the “+” and “/”, so the process is transferred to this one.
answer = 13 – (-20) * (4 + 6 / 3) + 5
The calculations in brackets are performed.
answer = 13 – (-20) * (4 + 2.0) + 5
It is read until the next ‘+’. The “*” has a higher priority and is therefore processed first.
answer = 13 – (-20) * (6.0) + 5
answer = 13 – (-120.0) + 5
‘-‘ and ‘+’ have the same priority, so ‘-‘ is processed.
answer = 13 – (-120.0) + 5
answer = 133.0 + 5
Further reading the formula on the right.
answer = 138.0
Finally, the assignment statement with the lowest priority is processed and the “variable” and “value” are linked.
That is all for this issue.
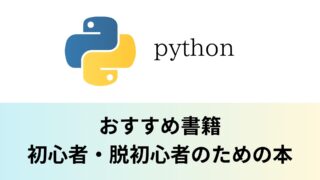
ブックマークのすすめ
「ほわほわぶろぐ」を常に検索するのが面倒だという方はブックマークをお勧めします。ブックマークの設定は別記事にて掲載しています。

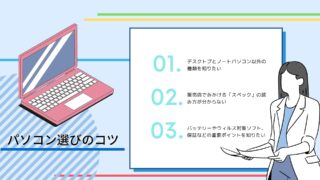