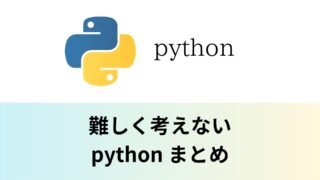
目標(Objectives)
「match文」について理解する。(To understand “match statement”.)
「リテラルパターン」について理解する(To understand Literal Patterns.)
「シーケンスパターン」について理解する(To understand Sequence Patterns.)
「マッピングパターン」について理解する(To understand Mapping Patterns.)
「ワイルドカードパターン」について理解する(To understand Wild Card Pattern.)
「キャプチャパターン」について理解する(To understand Capture Patterns.)
「バリューパターン」について理解する(To understand Value Patterns.)
詳しくはこちら:PEP 634 – Structural Pattern Matching: Specification
構造的パターンマッチング(match文)
Structural Pattern Matching(match statement)
「match文」は他の言語の「switch文」に似ていますが、パターンマッチングできるオブジェクトに「リスト」や「タプル」などのシーケンスも含まれます。更に、オブジェクトの持つ属性の型や個数のパターンも分岐に利用できます。
The “match statement” is similar to the “switch statement” in other programming languages, but it includes sequences such as “lists” and “tuples” among the objects that can be pattern matched. Furthermore, patterns in the type and number of attributes of objects can also be used for branching.
選択構造(match文)
Selection structure (match statement)
基本制御構造の復習
Review of basic control structures
基本制御構造とは(再掲載)
What is basic control structure (repetition)
「制御構造」のうち、基本とする3つの構造として「順次構造」「選択構造」「反復構造」が存在します。これらは「基本制御構造」と呼ばれて、次の構造をもっています。
- 「順次構造」…プログラムを書かれた順番で実行します。
- 「選択構造」…条件の成立・不成立によって実行するプログラムを選択します。
- 「反復構造」…条件の成立・不成立によって実行するプログラムを反復します。
Among the “control structures,” there are three basic structures: sequential, selective, and repetitive. These are called “basic control structures” and have the following structure.
- Sequential structure: Programs are executed in the order in which they are written.
- Selective structure: The program is selected to be executed according to whether the condition is satisfied or not.
- Iterative structure: The program to be executed is iterated according to whether the condition is satisfied or not.
「match文」の記述とその動き
Description of “match statement” and its movement
「match文」の記述
Description of “match statement”
「match文」は次のように記述します。下のイラストには記載していませんが、比較させるオブジェクトに「それ以外のオブジェクト」を指定する場合は「_(アンダースコア)」を利用します。
The “match statement” is described as follows. Although not shown in the illustration below, “_ (underscore)” is used to specify “other objects” as the object to be compared.
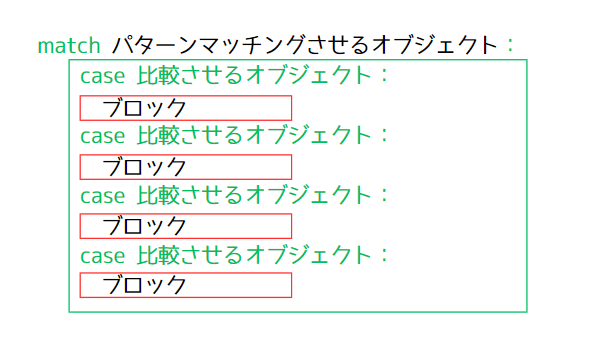
【English translation of captcha】
パターンマッチングされるオブジェクト
→Objects to be pattern matched
比較されるオブジェクト
→Contents of object being compared
ブロック
→Block
「match」の動き(1)/ The “match” movement (1)
変数「Objects_to_be_pattern_matched」にcaseで比較する値を指定すると、其々のブロックが処理されます。
If the variable “Objects_to_be_pattern_matched” is set to a value to be compared within a case, the corresponding block is processed.
Objects_to_be_pattern_matched = 1
match Objects_to_be_pattern_matched:
case 1:
print('int型の「1」です。')
case 3.14:
print('float型の「3.14」です。')
case "あいうえお":
print('str型の「あいうえお」です。')
case [1, 2, 3, 4, 5]:
print('list型の「[1, 2, 3, 4, 5]」です。')
case (int(), int(), int()):
print('要素数3,要素が全てint型のタプルです。')
case (float(), float(), int()):
print('要素数3,要素がfloat型、float型、int型のタプルです。')
case {"one":1, "two":2, "three":3}:
print('dict型の「{"one":1, "two":2, "three":3}」です。')
case _:
print('どれにも当てはまりません。')
【English translation of Japanese input part】
int型の「1」です
→It is “1” of type int
float型の「3.14」です。
→”3.14″ of type float
“あいうえお”:
→”aiueo”:.
str型の「あいうえお」です。
→type “aiueo” of type str
list型の「[1, 2, 3, 4, 5]」です。
→It is “[1, 2, 3, 4, 5]” of type list.
要素数3,要素が全てint型のタプルです。
→tuple with 3 elements and all elements of type int.
要素数3,要素がfloat型、float型、int型のタプルです。
→tuple with 3 elements and elements of type float, float, and int.
dict型の「{“one”:1, “two”:2, “three”:3}」です。
→It is a dict type “{“one”:1, “two”:2, “three”:3}”.
どれにも当てはまりません。
→None of the above.
実行結果:変数「Objects_to_be_pattern_matched」が「1」のとき。
Execution result: When the variable “Objects_to_be_pattern_matched” is “1”.

実行結果:変数「Objects_to_be_pattern_matched」が「3.14」のとき。
Execution result: When the variable “Objects_to_be_pattern_matched” is “3.14”.

実行結果:変数「Objects_to_be_pattern_matched」が「あいうえお」のとき。
Execution result: When the variable “Objects_to_be_pattern_matched” is “aiueo”.

実行結果:変数「Objects_to_be_pattern_matched」が「[1, 2, 3, 4, 5]」のとき。
Execution result: When the variable “Objects_to_be_pattern_matched” is “[1, 2, 3, 4 ,5]”.

実行結果:変数「Objects_to_be_pattern_matched」が「(1, 2, 3)」のとき。
Execution result: When the variable “Objects_to_be_pattern_matched” is “(1, 2, 3)”.

実行結果:変数「Objects_to_be_pattern_matched」が「(1.1, 2.2, 3)」のとき。
Execution result: When the variable “Objects_to_be_pattern_matched” is “(1.1, 2.2, 3)”.

実行結果:変数「Objects_to_be_pattern_matched」が「{“one”:1, “two”:2, “three”:3}」のとき。
Execution result: When the variable “Objects_to_be_pattern_matched” is “{“one”:1, “two”:2, “three”:3}”.

実行結果:caseに「_(アンダースコア)」を利用して。それ以外の値の時の処理を行わせることが可能です。(例:Objects_to_be_pattern_matchedに55を指定して実行)。
Execution result: Using “_ (underscore)” for “case”. It is possible to have the processing performed when the value is other than that. (e.g.: Executed with 55 specified for Objects_to_be_pattern_matched).

「match」の動き(2)/ The “match” movement (2)
キャプチャパターンを利用するとcaseの後に指定した変数にオブジェクト(リテラル等も含む)を結びつけることができます。
The capture pattern can be used to bind an object (including literals, etc.) to a variable specified after the “case”.
Objects_to_be_pattern_matched = 55
match Objects_to_be_pattern_matched:
case 1:
print('int型の「1」です。')
case 3.14:
print('float型の「3.14」です。')
case Capture_variable:
print('キャプチャパターンではcaseに指定した変数「Capture_variable」に値「{}」を束縛します。'.format(Capture_variable))
print('match文のブロックの外でCapture_variableを呼び出すと--->', Capture_variable)
【English translation of Japanese input part】
キャプチャパターンではcaseに指定した変数「Capture_variable」に値「{}」を束縛します。’.format(Capture_variable)
→The capture pattern binds the value “{}” to the variable “Capture_variable” specified in the case.
※The “{}” is a replacement field into which the value specified by the format method is entered.
match文のブロックの外でCapture_variableを呼び出すと
→When you call Capture_variable outside of a block of match statements
実行結果:3つ目の「Capture_variable」に「55」が束縛されています。
Execution result: “55” is bound to the third “Capture_variable”.

Objects_to_be_pattern_matched = 55
match Objects_to_be_pattern_matched:
case 1:
print('int型の「1」です。')
case 3.14:
print('float型の「3.14」です。')
case Capture_variable:
print('キャプチャパターンではcaseに指定した変数「Capture_variable」に値「{}」を束縛します。'.format(Capture_variable))
# 次を記述すると「Capture_variable」部分でエラーとなります。
case "あいうえお":
print('str型の「あいうえお」です。')
print('match文のブロックの外でCapture_variableを呼び出すと--->', Capture_variable)
キャプチャパターンでは変数に値が結びつく場合、ディープコピーとなります。
In the capture pattern, values are bound to variables in deep copy.
Objects_to_be_pattern_matched = [1, 2, [1, 2]]
match Objects_to_be_pattern_matched:
case 1:
print('int型の「1」です。')
case 3.14:
print('float型の「3.14」です。')
case Capture_variable:
print('キャプチャパターンではcaseに指定した変数「Capture_variable」に値「{}」を束縛します。'.format(Capture_variable))
print('match文のブロックの外でCapture_variableを呼び出すと--->', Capture_variable)
Objects_to_be_pattern_matched = [1, 2, [1, 3]]
print('match文のブロックの外でCapture_variableを呼び出すと--->', Capture_variable)
実行結果
Execution result

「match」の動き(3)/ The “match” movement (3)
定数などを利用する場合バリューパターンでパターンマッチングさせます。
When using constants, etc., pattern matching is performed with a value pattern.
ファイル「CONST.py」を作成して次用のように入力します。
Create the file “CONST.py” and enter the following.
const_val_1 = 1
const_val_10 = 10
別のファイルから「CONST.py」を呼び出してmatch文で利用します。
Call “CONST.py” from another file and use it in a match statement.
import CONST
Objects_to_be_pattern_matched = 10
match Objects_to_be_pattern_matched:
case CONST.const_val_1:
print('int型の「1」です。')
case CONST.const_val_10:
print('int型の「10」です。')
case _:
print('どれにも当てあはまりません。')
実行結果
Execution Result

今回は以上になります。
That’s all for this time.
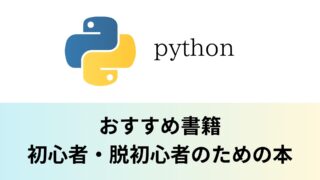
ブックマークのすすめ
「ほわほわぶろぐ」を常に検索するのが面倒だという方はブックマークをお勧めします。ブックマークの設定は別記事にて掲載しています。

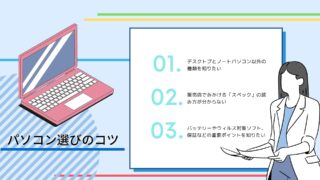