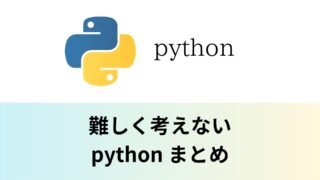
Objective
To understand how to use “variables”.
In the previous article, we dealt with an overview of “variables” in python. This time, we will deal with the basic usage of variables. The way “variables” are used is not always as simple as in this article (Python (Part.6) | python [Using Variables]), but by understanding what they can do, you will be able to apply them to your work.
Python Basics (Using Variables)
Defining Variables
Variables can be used by defining them. The word “define” means not only giving a name to a variable, but also assigning a value to it using an assignment statement (“=”) to complete the definition. Below is a scene of an actual definition.
int_num = 5
str_name = 'ほわほわ'
list_obj1 = [1, 2, 3, 4, 5]
At this time, the “ID (identification number)” in each value is assigned to the “variable” and tied to the “value”. (The figure below is re-posted.)
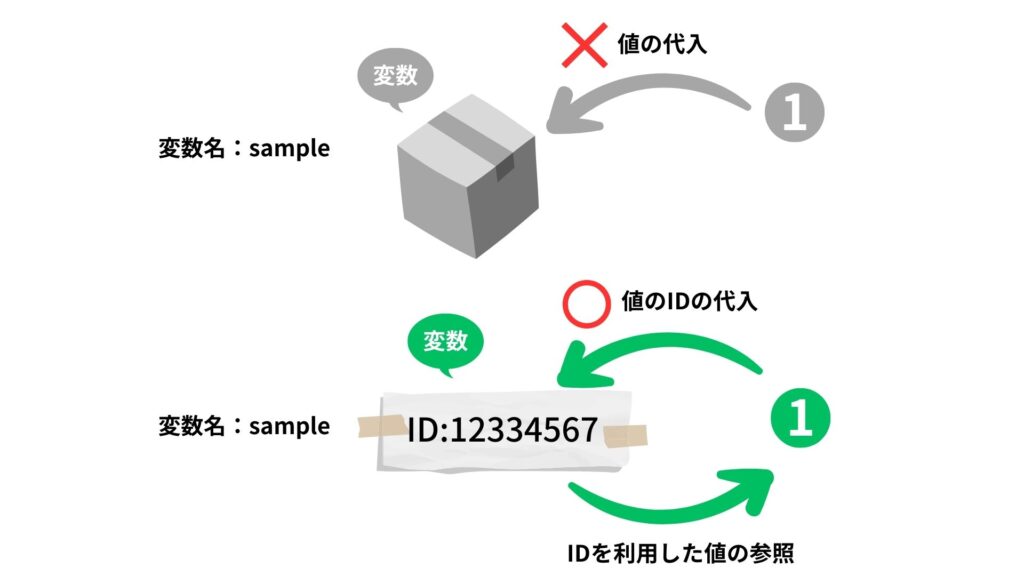
【English translation of captcha】
変数 → Variables
値の代入 → Value Assignment
値のIDの代入 → Assigning an ID to a value
IDを利用した値の参照 → Referencing a value by its ID
How to name “variables“
How to name “variables“
Defining a variable requires two tasks: naming the variable and associating values with it. In this section, we would like to review the rules for naming variables. The following rules apply to naming variables
- Available characters are as follows
- 「a」~「z」、「A」~「Z」、「0」~「9」、「_(Underscore)」「Kanji」
- Capital letters and lowercase letters are distinguished.
- How to give a name that is not preferred
- Although “_ (underscore)” can be used as the first character, it is not often used as the first character in variables in “python” because “_ (underscore)” is used as the first character for special purposes such as methods and encapsulation.
- As a rule, several patterns cannot be used for variable names.
- Numeric characters in the first letter (1name, 2tel, 3,address, etc.)
- Reserved words (expressions and statements prepared for programming in python, such as “if” and “for”)
How to name ”variables” for use as ”constants”
Since constants are not supported in python, variables named with all “uppercase letters” and “_ (underscore)” are used as constants as a convention.
CONST_ID = 5
CONST_STR = 'IT'
How to use “Variables“
Variables are used to reuse values. For this reuse, it is necessary to associate a “value” with a “variable,” and the assignment statement “=” is used to write this operation in a program. Variables can be used in the following ways. All of them are frequently used.
Simple stringing
This is a one-to-one association of “variables” and “values.
example_variable_1 = 55
example_variable_2 = 3.14
example_variable_3 = 'sample'
example_variable_4 = True
example_variable_5 = [1, 2, 3, 4, 5]
Simply write “variable,” “assignment statement,” and “value” in that order.
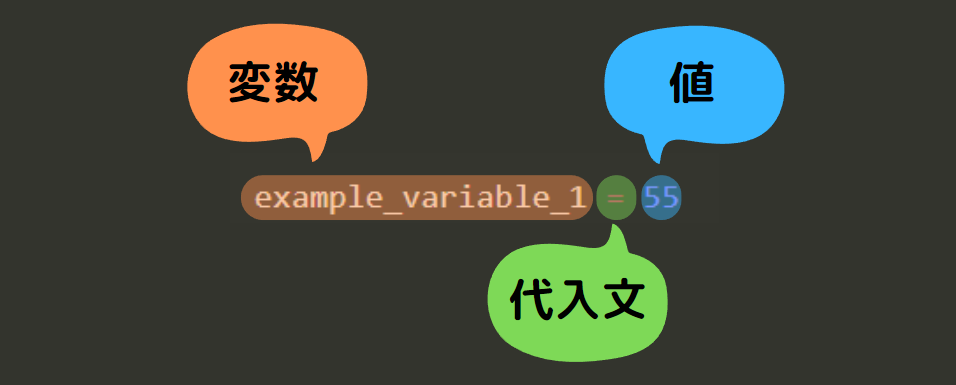
【English translation of captcha】
変数 → Variables
代入文 → Assignment statement
値 → Value
How to tie a single value to multiple variables simultaneously
This is a method of simultaneously linking “values” by arranging “multiple variables” using an “assignment statement”.
example_variable_1 = example_variable_2 = 55
In the above program, the two variables will refer to “55”.
How to tie individual values to individual variables simultaneously
On the left side of the assignment expression, “multiple variables” are separated by “, (comma)” and arranged in order from left to right, and on the right side, “values to be associated” with the variables on the left side are separated by “, (comma)” and arranged in the same order as the variables.
example_variable_1, example_variable_2, example_variable_3 = 55, 3.14, 'sample'
As shown in the capture below, each variable and each value is separated by “,” (comma).
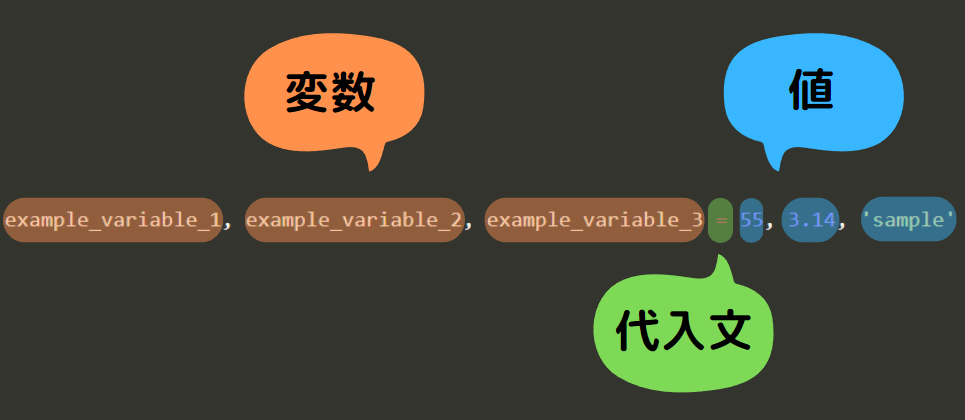
【English translation of captcha】
変数 → Variables
代入文 → Assignment statement
値 → Value
The “value” portion can also use “arithmetic operations“.
example_variable_1, example_variable_2, example_variable_3 = 55 + 5, 3.14 * 2 * 2, 'sample'
As we will cover in the future on this site, variables can also be associated with “instances of classes” and “return values of functions”. That is all for this time.
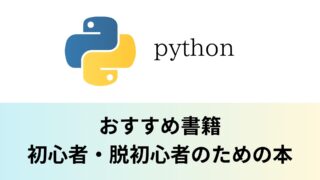
ブックマークのすすめ
「ほわほわぶろぐ」を常に検索するのが面倒だという方はブックマークをお勧めします。ブックマークの設定は別記事にて掲載しています。

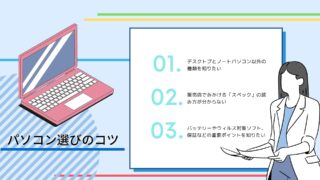