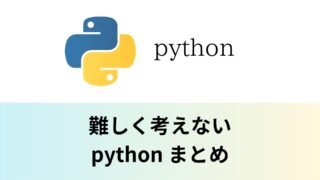
python| まとめ | 現役エンジニア&プログラミングスクール講師「python」のまとめページです。pythonに関して抑えておきたい知識や文法やにについて記事をまとめています。まとめページの下部には「おすすめの学習書籍」「おすすめのITスクール情報」「おすすめ求人サイト」について情報を掲載中...
目標
- 注文された商品の個数を修正する機能とキャンセルする機能を実装する
- 会計完了ボタンを設置して会計の処理を完了させる機能を実装する。
レジ管理アプリケーションの作成
レジ管理アプリケーションの作成(再掲載)
ここからは、商店スタッフが利用するためのレジ管理アプリケーションを作成していきます。具体的には、全てのテーブル状況を確認できたり、各テーブルの注文状況を確認でき、ユーザー側では行えない注文済みの商品の取り消しや、会計を完了させる機能を実装します。
レジ管理アプリケーションのプログラミング
models.pyの編集(teblesapp内)
models.pyファイルを次のように編集します。
from apps.common.db import db
# テーブルモデル
class Table(db.Model):
id = db.Column(db.Integer, primary_key=True)
number = db.Column(db.Integer)
# 伝票(Bill)とのリレーション
bill = db.relationship(
"Bill", back_populates="table"
) # 複数の伝票が1つのテーブルに紐づく
def __repr__(self):
return f"<Table {self.number}>"
これ以降では、テーブルは会計ごとに新しい伝票と結びつくようにプログラムを修正していきます。Tableモデルに大きな変化はありませんが、コメントの部分を「複数の伝票が1つのテーブルに紐づく」としています。
テーブルは、複数の伝票と結びつくためルートのプログラムで「table.bill」はリストとして機能します。このうち最新の伝票をアクティブにするので、その取得方法は、スライス式を利用して、table.bill[ -1 ]で取得します。
app.pyの編集(ordersapp内)
app.pyファイルを次のように編集します。
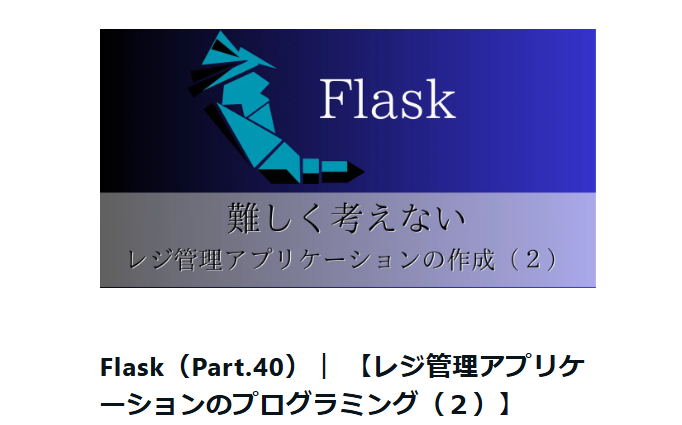
今回は以上になります。
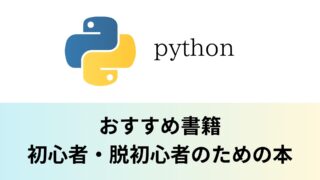
「python」おすすめ書籍 ベスト3 | 現役エンジニア&プログラミングスクール講師「python」の学習でお勧めしたい書籍をご紹介しています。お勧めする理由としては、考え方、イメージなどを適切に捉えていること、「生のpython」に焦点をあてて解説をしている書籍であることなどが理由です。勿論、この他にも良い書籍はありますが、特に質の高かったものを選んで記事にしています。ページの下部には「おすすめのITスクール情報」「おすすめ求人サイト」について情報を掲載中。...
ブックマークのすすめ
「ほわほわぶろぐ」を常に検索するのが面倒だという方はブックマークをお勧めします。ブックマークの設定は別記事にて掲載しています。

「お気に入り」の登録・削除方法【Google Chrome / Microsoft Edge】「お気に入り」の登録・削除方法【Google Chrome / Microsoft Edge】について解説している記事です。削除方法も掲載しています。...
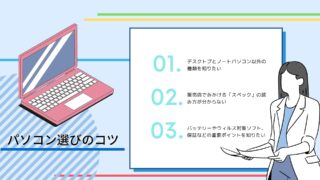
【パソコン選び】失敗しないための重要ポイント | 現役エンジニア&プログラミングスクール講師【パソコン選び】失敗しないための重要ポイントについての記事です。パソコンのタイプと購入時に検討すべき点・家電量販店で見かけるCPUの見方・購入者が必要とするメモリ容量・HDDとSSDについて・ディスプレイの種類・バッテリーの持ち時間や保証・Officeソフト・ウィルス対策ソフトについて書いています。...
Blueprint Flask Flask-SQLAlchemy Jinja2 MVT python session SQLite アップロード エンジニア セッション テンプレートエンジン バリデーション フレームワーク ルーティング 作成方法 初心者 利用方法 注意点 画像 統合
この続きはNoteとなります。